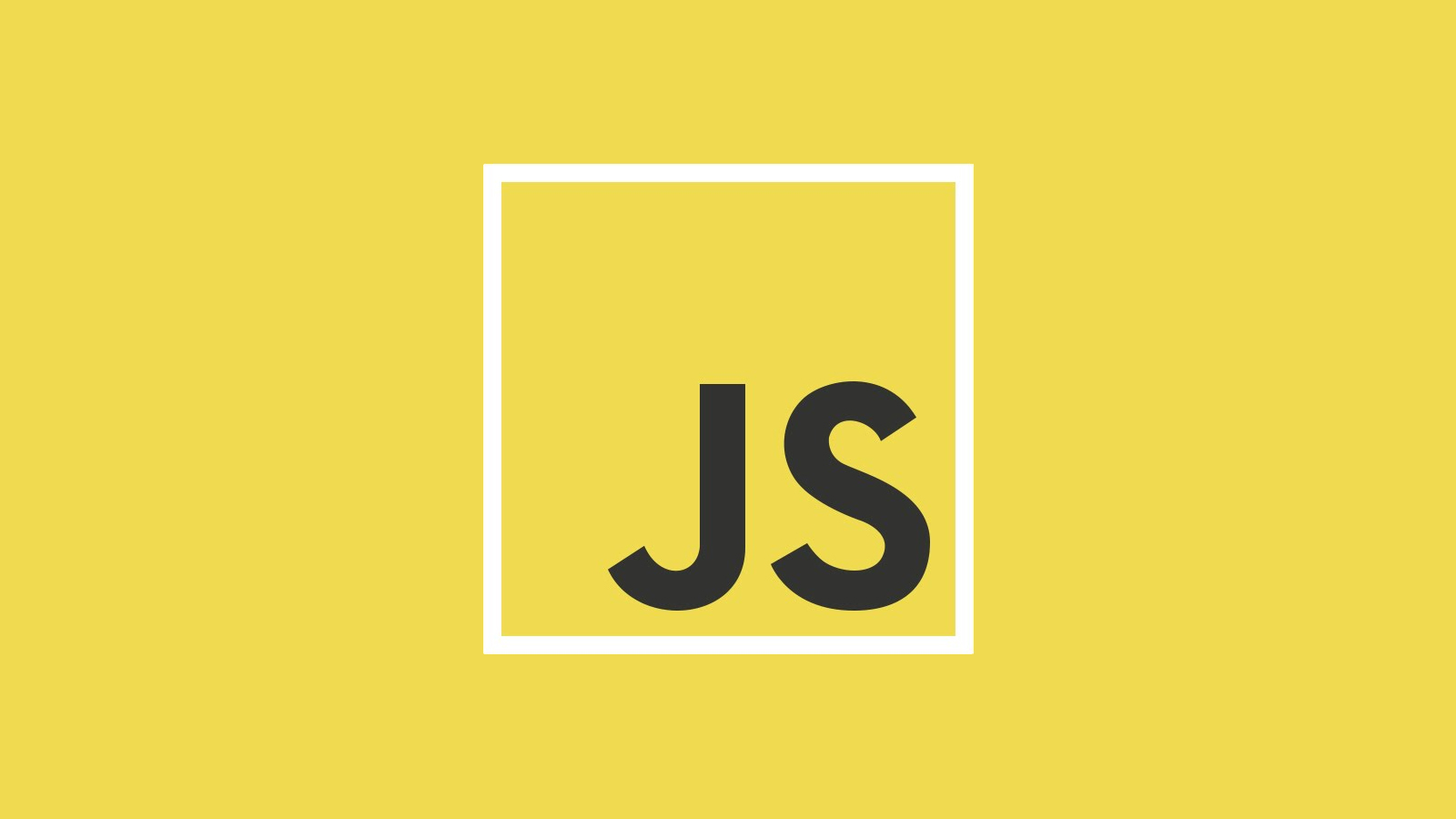
Async / Await
Interested in ReactJS, RxJS and ReasonML.
ES2017(ECMA-262)
μμ μλ‘κ² μΆκ°λ async/await
λ λΉλκΈ° ν¨μλ₯Ό μ²λ¦¬νκΈ° μν ν€μλμ΄λ€.
μ²μ μ μλ κ²μ TypeScript 1.7μλ€.
λν, async/await
λ Promise
μμ ꡬμΆλμκ³ , κΈ°μ‘΄μ λͺ¨λ νλ‘λ―Έμ€ κΈ°λ° APIμ νΈνλκΈ° λλ¬Έμ νλ‘λ―Έμ€μ κ°μ΄ μ¬μ©νκ±°λ, νλ‘λ―Έμ€λ₯Ό λ체ν μ μλ€.
λΉλκΈ° λμμ μν λκΈ°μ ꡬ문μ μ 곡ν¨μΌλ‘μ¨ μ½λ κ°λ
μ±μ λμΌ μ μμΌλ©°, μλ¬ νΈλ€λ§μ μν΄ try/catch
λ₯Ό μ¬μ©νλ€.
μμνκΈ° μ μ
async
μ await
λ ν€μλμ΄λ―λ‘ polyfill
μ΄ μμΌλ©°, μ΄μ λΈλΌμ°μ λ₯Ό μ§μνλ €λ©΄, babel
λ± νΈλμ€νμΌλ¬λ₯Ό μ¬μ©ν΄μΌ νλ€.
μ¬μ©νλ λΈλΌμ°μ κ° async
, await
λ₯Ό μ§μνλμ§ νμΈνλ €λ©΄, Can I use?μμ ν΄λΉ ν€μλλ₯Ό κ²μνλ©΄ λλ€.
μμ μ½λλ₯Ό ν΅ν΄ async/await μ΄ν΄λ³΄κΈ°
function willBeAwaited() {
return new Promise(function (resolve) {
setTimeout(resolve, 400, 42);
});
}
async function asyncFunc () {
const resolvedValue = await willBeAwaited();
console.log(resolvedValue);
return resolvedValue + 500;
}
asyncFunc()
.then(function (resolvedValue) {
console.log(resolvedValue);
});
// 42
// 542
async
ν€μλλ μΌλ°μ μΈ ν¨μλ₯Ό μλμΌλ‘ νλ‘λ―Έμ€ ννλ‘ λ§λ€λ©°, async
ν¨μλ₯Ό νΈμΆνλ©΄ ν΄λΉ ν¨μκ° λ¬΄μμ λ°ννλ resolve
λλ€. λν, async
ν¨μλ await
μ μ¬μ©μ κ°λ₯νκ² νλ€.
await
ν€μλλ νλ‘λ―Έμ€ κ°μ²΄μ μλνλ©°, ν¨μμ νΈμΆ μμ μ¬μ©λλ©΄ νλ‘λ―Έμ€κ° ν΄κ²°λ λκΉμ§ μ½λ μ€νμ μ€λ¨νκ² νλ€. await
λ μ€μ§ async
ν¨μμ μ¬μ©λ μ μλ€.
asyncFunc
λ₯Ό μΌλ° νλ‘λ―Έμ€ μ€νμΌλ‘ μ¬μμ±νλ©΄, λ€μκ³Ό κ°λ€.
function asyncFunc() {
return willBeAwaited()
.then((resolvedValue) => {
console.log(resolvedValue);
return resolvedValue + 500;
});
}
μλ¬ νΈλ€λ§
νλ‘λ―Έμ€λ ν΄κ²°(resolve
)λ μλ μκ³ , κ±°μ (reject
)λ μ μμΌλ©°, κ±°μ λλ€λ©΄ νλ‘λ―Έμ€ κ°μ²΄μ Promise.prototype.catch
λ©μλλ₯Ό ν΅ν΄ μ²λ¦¬νλ€.
async/await
μ κ²½μ°μλ try { ... } catch { ... }
λ₯Ό μ¬μ©νλ€.
function willBeAwaited() {
return new Promise(function (resolve, reject) {
setTimeout(reject, 400, 'Error occured!');
});
}
async function asyncFunc () {
try {
const resolvedValue = await willBeAwaited();
console.log(resolvedValue);
return resolvedValue + 500;
} catch (e) {
console.log(e);
return 'Error occured when \'willBeAwaited\' is executed';
}
}
asyncFunc()
.then(function (resolvedValue) {
console.log(resolvedValue);
});
// Error occured!
// Error occured when 'willBeAwaited' is executed
λ§μ½, async
ν¨μμμ κ±°μ λ νλ‘λ―Έμ€λ₯Ό λ°ννλ €λ©΄ throw
λ₯Ό ν΅ν΄ μλ¬λ₯Ό λμ§λ©΄ λλ€.
function willBeAwaited() {
return new Promise(function (resolve, reject) {
setTimeout(reject, 400, 'Error occured!');
});
}
async function asyncFunc () {
try {
const resolvedValue = await willBeAwaited();
console.log(resolvedValue);
return resolvedValue + 500;
} catch (e) {
console.log(e);
throw new Error(e);
}
}
asyncFunc()
.then(function (resolvedValue) {
console.log(resolvedValue);
})
.catch(function (e) {
console.log(e);
});
// Error occured!
// Error occured!
async/awaitμ νκ³
1. async
κ° μμ΄λ await
λ₯Ό μ¬μ©ν μ μλ€.
μ μ μ€μ½νλ μΌλ° ν¨μ μ€μ½ν μμμ await
λ₯Ό μ§μ μ¬μ©ν μ μμμ μλ―Ένλ€.
async function one() {
return 1;
}
// const num = await one();
// can not be executed
async function main() {
const num = await one();
console.log(num);
}
main();
2. Promise.all
κ³Ό κ°μ κ²½μ°λ₯Ό λ체ν μ μλ€.
λͺ¨λ λΉλκΈ° νΈμΆμ΄ λλ λκΉμ§ κΈ°λ€λ €μΌ νλ κ²½μ°, Promise.all
μ μ¬μ©νλ κ²μ΄ ν¨μ¬ μ’λ€.
function sleep(ms = 0) {
return new Promise(resolve => setTimeout(function () {
resolve(ms);
}, ms));
}
(async function awaitAyncFunctions() {
const start = Date.now();
await sleep(1000);
await sleep(3000);
await sleep(2000);
const end = Date.now();
console.log(`execution time is ${Math.round((end - start) / 1000)}seconds.`);
// execution time is 6seconds.
})();
(async function awaitAyncFunctions() {
const start = Date.now();
const results = await
Promise.all([sleep(1000), sleep(3000), sleep(2000)]);
const end = Date.now();
console.log(`execution time is ${Math.round((end - start) / 1000)}seconds.`);
// execution time is 3seconds.
})();